接上次分享的自定义cell进行了优化:
指定根视图:
- self.window.rootViewController = [[UINavigationController alloc] initWithRootViewController:[[RootTableViewController alloc] initWithStyle:UITableViewStylePlain]];
RootTableViewController.m
- #import "WGModel.h"
- #import "WGCell.h"
-
- @interface RootTableViewController ()
-
- @property (nonatomic, strong) NSMutableDictionary *dataDict;
-
- @end
-
- @implementation RootTableViewController
-
- - (void)viewDidLoad
- {
- [super viewDidLoad];
-
- self.dataDict = [NSMutableDictionary dictionary];
-
- [self.tableView registerClass:[WGCell class] forCellReuseIdentifier:@"cell"];
- [self loadDataAndShow];
- }
请求数据: - - (void)loadDataAndShow
- {
- NSURL *url = [NSURL URLWithString:@"http://api.breadtrip.com/trips/2387133727/schedule/"];
- NSURLRequest *request = [NSURLRequest requestWithURL:url];
- [NSURLConnection sendAsynchronousRequest:request queue:[NSOperationQueue mainQueue] completionHandler:^(NSURLResponse *response, NSData *data, NSError *connectionError) {
-
- if (data != nil) {
- NSArray *array = [NSJSONSerialization JSONObjectWithData:data options:NSJSONReadingAllowFragments error:nil];
- for (NSDictionary *dict in array) {
- NSString *key = dict[@"date"];
- NSArray *placesArray = dict[@"places"];
- NSMutableArray *mutableArray = [NSMutableArray array];
- for (NSDictionary *placesDict in placesArray) {
- WGModel *model = [[WGModel alloc] init];
- [model setValuesForKeysWithDictionary:placesDict];
- model.isShow = NO;
- [mutableArray addObject:model];
- }
- [self.dataDict setObject:mutableArray forKey:key];
- }
- [self.tableView reloadData];
- }
-
-
- }];
- }
#pragma mark - Table view data source
- - (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
- {
- return self.dataDict.allKeys.count;
- }
-
- - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
- {
- NSString *key = self.dataDict.allKeys[section];
- return [self.dataDict[key] count];
- }
-
-
- - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
- {
- WGCell *cell = [tableView dequeueReusableCellWithIdentifier:@"cell" forIndexPath:indexPath];
-
- NSString *key = self.dataDict.allKeys[indexPath.section];
- NSMutableArray *mutableArray = self.dataDict[key];
- WGModel *model = mutableArray[indexPath.row];
- [cell configureCellWithModel:model];
-
- if (model.isShow == YES) {
- [cell showTableView];
- } else {
-
- [cell hiddenTableView];
- }
-
- return cell;
- }
自适应高 - - (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
- {
- NSString *key = self.dataDict.allKeys[indexPath.section];
- NSMutableArray *mutableArray = self.dataDict[key];
- WGModel *model = mutableArray[indexPath.row];
- if (model.isShow) {
- return (model.pois.count + 1) * 44;
- } else {
- return 44;
- }
- }
点击cell会走的方法 - - (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
- {
- NSString *key = self.dataDict.allKeys[indexPath.section];
- NSMutableArray *mutableArray = self.dataDict[key];
- WGModel *model = mutableArray[indexPath.row];
- model.isShow = !model.isShow;
- [self.tableView reloadRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationAutomatic];
- }
自定义cell -
- #import <UIKit/UIKit.h>
- @class WGModel;
- @interface WGCell : UITableViewCell<UITableViewDataSource, UITableViewDelegate>
-
- @property (nonatomic, strong) UILabel *aLabel;
- @property (nonatomic, strong) UITableView *tableView;
-
-
- - (void)configureCellWithModel:(WGModel *)model;
-
- - (void)showTableView;
- - (void)hiddenTableView;
-
- @end
-
-
- #import "WGCell.h"
- #import "WGModel.h"
-
- @interface WGCell ()
-
- @property (nonatomic, strong) NSMutableArray *dataArray;
-
- @end
-
- @implementation WGCell
-
-
- - (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier
- {
- if (self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]) {
- self.dataArray = [NSMutableArray array];
- [self addAllViews];
- }
- return self;
- }
-
- - (void)addAllViews
- {
- self.aLabel = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, [UIScreen mainScreen].bounds.size.width, 44)];
- self.aLabel.backgroundColor = [UIColor greenColor];
- [self.contentView addSubview:self.aLabel];
- self.tableView = [[UITableView alloc] initWithFrame:CGRectMake(0, 44, [UIScreen mainScreen].bounds.size.width, 0) style:UITableViewStylePlain];
-
- self.tableView.delegate = self;
- self.tableView.dataSource = self;
- [self.tableView registerClass:[UITableViewCell class] forCellReuseIdentifier:@"testCell"];
-
- }
-
- - (void)showTableView
- {
- [self.contentView addSubview:self.tableView];
- }
-
- - (void)hiddenTableView
- {
- [self.tableView removeFromSuperview];
- }
-
- - (void)configureCellWithModel:(WGModel *)model
- {
- [self.dataArray removeAllObjects];
- self.aLabel.text = model.place[@"name"];
-
- NSArray *array = model.pois;
- for (NSDictionary *dict in array) {
- NSString *str = dict[@"name"];
- [self.dataArray addObject:str];
- }
- CGRect frame = self.tableView.frame;
- frame.size.height = 444 * array.count;
- self.tableView.frame = frame;
- [self.tableView reloadData];
-
- }
-
-
- - (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
- {
- return 1;
- }
-
- - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
- {
- return self.dataArray.count;
- }
-
- - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
- {
- UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"testCell" forIndexPath:indexPath];
- NSString *str = self.dataArray[indexPath.row];
- cell.textLabel.text = str;
- return cell;
- }
准备一个model类 -
- #import <Foundation/Foundation.h>
-
- @interface WGModel : NSObject
-
- @property (nonatomic, assign) BOOL isShow;
- @property (nonatomic, strong) NSDictionary *place;
- @property (nonatomic, strong) NSArray *pois;
-
- @end
-
-
-
- #import "WGModel.h"
-
- @implementation WGModel
-
- - (void)setValue:(id)value forUndefinedKey:(NSString *)key
- {
-
- }
-
- @end
最终效果: 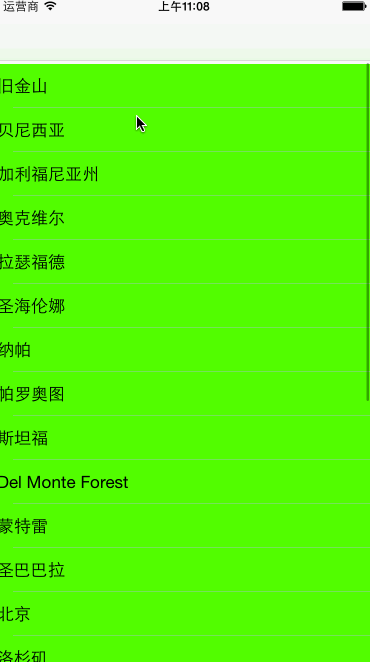
每日更新关注: 新浪微博
原文地址:http://blog.csdn.net/qq_31810357/article/details/49847711